Programming in
Visual Basic
Working with Text
:
Text boxes, as the name
implies, are designed to work directly
with text. All text 'strings' involved
within a program have to be enclosed
within quotes.
For example, to assign the value
"Radio" to the Item text box
within the Order Entry Form, we would use
the following code:
txtItem = "Radio"
Task
Four :
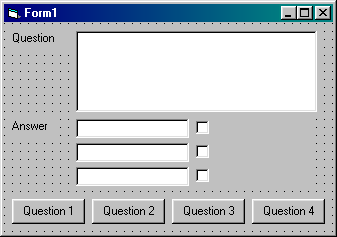
1. Load 'QUIZ.VBP' and
add 4 command buttons with captions
'Question 1', 'Question 2', 'Question 3',
'Question 4' and names cmdQ1, cmdQ2,
cmdQ3, cmdQ4.
2. Set the Multiline property of the
txtQuestion text box to True. This will
allow long questions to word wrap.
3. Double click on the Question 1 command
button and enter the following code:
Sub cmdQ1_Click ()
txtQuestion
= "What do you call a small
piece of silicon which has a
number of electronic circuits
fabricated on it?"
txtAnswer1
= "Byte"
txtAnswer2
= "Chip"
txtAnswer3
= "Port"
End Sub
Click on
the Start Program button, click on the
command button to see the question being
displayed. Click on the End Program
button when you've finished.
Exercise Four :
1. Enter code for the
Question 2, Question 3, Question 4
command buttons using the following:
Question 2
|
A keyboard is used to
|
Answers
|
Output data
Input data
Control input devices
|
Question 3
|
Which of the following is not
an output device?
|
Answers
|
Light pen
Plotter
VDU
|
Question 4
|
The programs that run on a
computer are known as
|
Answers
|
Hardware
Liveware
Software
|
2. Check
that all questions and answers are
displayed correctly.
Making
Decisions :
You can include decision
structures in any event procedure. A
decision structure will test a condition
and then perform operations depending on
whether or not the condition is met or
not (True or Flase). A condition usually
makes a comparison between two values
using one of the following operators:
Operator
|
Meaing
|
Examples
|
Explanation
|
=
|
Equal to
|
txtItem ="Radio"
|
is the item a Radio
|
<>
|
Not Equal to
|
txtPrice <> 0
|
is the price not 0
|
>
|
Greater than
|
txtPrice > 12.54
|
is the price greater than
12.54 £
|
<
|
Less than
|
txtPrice < 10.00
|
is the price less than 10.00
£
|
In Visual
Basic, the If...Then...Else...End if
structure is used to make decisions. The
If, Then and End if parts of the
structure are compulsory.
The Else statement is only used when an
alternative set of operationsneed to be
carried out if the original condition is
not met.
Examples :
To set the Price to
£12.54 if the item is a radio:
If txtItem =
"Radio" Then
txtPrice =
12.54
End If
To set the
address details if the name is P Brown:
If txtName = "P
Brown" Then
txtAddress1
= "East Birmingham
College"
txtAddress2
= "Garretts Green Lane"
txtTown =
"Birmingham"
txtPostCode
= "B33 0TS"
txtPhone =
"743 4471"
End If
To check
if the user has chosen the correct check
box for Question 1 in the quiz:
If chkAnswer2 = True Then
Score = 1
Else
Score = 0
End If
N.B.
Notice the use of indents to line up the
main parts of the stucture. This is used
extensively in Visual Basic to make the
program easier to follow and to make
checking for the start and end of a
particular structure easier.
Task Five :
1. Open the project
STOCK.VBP. Add another command button
with a caption of Find Item and a Name
cmdFindItem.
2. Double click on this command button
and add the following code:
Sub cmdFindItem_Click ()
If txtItem = "Radio"
Then
txtPrice =
12.54
End If
If txtItem =
"Headphones" Then
txtPrice =
23.5
End If
If txtItem = "Glasses"
Then
txtPrice =
13.99
End If
If txtItem = "Teddy
Bear" Then
txtPrice =
23.49
End If
End Sub
3. Click
on the Start Program button, enter a
valid Item e.g. Radio and click on the
Find Item command button to see the price
being displayed. Try the program with
other valid items (Headphones, Glasses or
Teddy Bear). What happens if you enter an
item that is not in the list? What
happens if you type radio instead of
Radio? What happens when you click on the
Calculate command button? (We'll come
back to this shortly)
4. Stop the program and re-save the
project.
Exercise Five :
1. Open the ADDRESS.VBP
project and add a Find Name command
button (cmdFindName)
2. Add code for this button consisting of
a number of If Then ... End If statements
to check for the following names and the
appropriate details from the table below:
Name
|
Address 1
|
Address 2
|
Town
|
Post Code
|
Phone
|
P Brown
|
E. Birmingham Coll.
|
Garretts Green Lane
|
Birmingham
|
B33 0TS
|
743 4471
|
J. Jones
|
1 New Street
|
Solihull West
|
Mids
|
B92 6TY
|
706 2234
|
B. Jaffer
|
33 Green Lane
|
Small Heath
|
Birmingham
|
B12 3ER
|
356 3434
|
C. Henry
|
243 Field Road
|
Sheldon
|
Birmingham
|
B26 4ER
|
742 2323
|
3. Open
the STOCK.VBP project and add a new
label, text box and command button as
shown below:
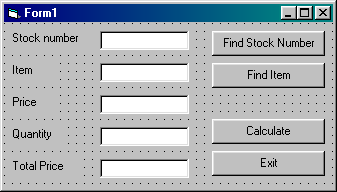
Text box name
|
txtStockNumber
|
Command button name
|
cmdFindNumber
|
4. Enter
code for this new command button to check
the Stock Number and update the text
boxes as below:
Stock Number
|
Item
|
Price
|
1000
|
Radio
|
12.54
|
1001
|
Headphones
|
23.50
|
1002
|
Glasses
|
13.99
|
1003
|
Teddy Bear
|
23.49
|
1004
|
Rocking Horse
|
45.98
|
1005
|
Dinner Set
|
24.99
|
Page [<<
PREV] 1 2
3 4 5
6 [NEXT
>>]
Back to Tutorials
- Main
|