Programming in
Visual Basic
Properties and
Names:
We had a quick look at
properties in Unit One when we set up the
Caption property for the labels and
command buttons. An important part of the
design of a Visual Basic project is to
ensure that the forms displayed for the
user are well labelled and provide
straightforward and validated data entry.
For the programmer, the use of meaningful
names for controls makes coding easier to
understand and debug. Trying to
understand and alter something like
If x1 > y1 Then
Text2 = "Too
much"
Else
z2 = 2
End If
is almost impossible. What are x1, y1 and
z2? What are we checking? Where is Text2
situated?
Properties:
For forms, labels and
command buttons, the Caption property
should always be set. The caption is the
text that appears on a label or command
button or at the top of a form.
For text boxes, the value
that you want to appear as the form is
loaded should always be set. In Unit One,
we didn't do this and the default values
of Text1, Text2, Text3 and Text4 were
displayed. This is unsatisfactory for
this application - the user would need to
delete this text before meaningful values
could be entered. Normally, we don't want
to anything to be displayedin data entry
boxes and would therefore clear the Text
property. Occasionally, we would set a
default value, for example, setting a VAT
box to 17.5%.
Names:
For controlsthat we need
to refer to during coding, the name
property needs to be set. This enables us
to write meaningful programs that are
easy to follow and easy to change. There
are a number of conventions used by
programmers, but control names should
always be descriptive and, if naming
controls in Visual Basic consists of a
standard prefix to describe the type of
control, followed by whatever you want to
call the object.
For example, in Unit One, we ended up
with a form called Form1 (the Order Entry
form), a text box called Text1 (the Data
Entry box for the Item Name) and a
command button called Command1. The
following names would meet the standard
Visual Basic naming convention:
Control
|
Control Prefix
|
Object Name
|
Complete Name
|
Form1
|
frm
|
Order
|
frmOrder
|
Text1
|
txt
|
Item
|
txtItem
|
Command1
|
cmd
|
Calculate
|
cmdCalculate
|
N.B. In
Visual Basic, object names cannot contain
spaces. Normally we use capitalisation
for multiple words. For Example, if we
wished to name the stock form "Stock
Data Entry" we would use the name
frmStockDataEntry.
Task 2:
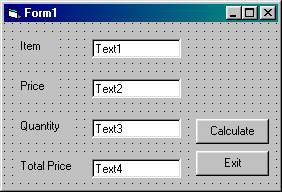
1. Open the project
created in Exercise One (File Menu, Open
Project, stock.vbp)
2. Click somewhere on the form's
background - not on any of the controls.
3. Click on the Caption property and
change the caption to 'Order Entry'.
4. Click on the Name property and change
the name to frmOrder
N.B. Note the difference between Caption
and Name. The caption is a label for the
form to help the eventual user understand
what this form does. We make this
descriptive and spaces are allowed. The
Name is the name used by tou, the
programmer. It is still descriptive, but
just enough to tell us the type of object
and an indication of its purpose. No
spaces are allowed.
5. Click on the text box Text1. In the
properties window, click on the name
property and change the name to txtItem.
Click on the text property and delete the
text 'Text1'. Similarly delete the
existing text and change the name
properties of Text2, Text3 and Text 4 to
txtPrice, txtQuantity and txtTotalPrice
respectively.
6. Change the name proerties of the two
command buttons to cmdCalculate and
cmdExit.
Click on the Save shortcut on the
toolbar to save your changes
(alternatively choose Save Project from
the File menu)
Exercise Two :
1. Open th Project
ADDRESS.VBP and make the following
changes to its properties:
Default Name/Caption
|
New Caption
|
New Name
|
Notes
|
Form1
|
Address Entry
|
|
|
Text1
|
|
txtName
|
Delete existing text
|
Text2
|
|
txtAddress1
|
Delete existing text
|
Text3
|
|
txtAddress2
|
Delete existing text
|
Text4
|
|
txtTown
|
Delete existing text
|
Text5
|
|
txtPostCode
|
Delete existing text
|
Text6
|
|
txtPhone
|
Delete existing text
|
Exit
|
|
cmdExit
|
|
2. Open
the Project QUIZ.VBP and make the
following changes to its properties:
Default Name/Caption
|
New Caption
|
New Name
|
Notes
|
Form1
|
Quiz
|
frmQuiz
|
|
Text1
|
|
txtQuestion
|
Delete existing text
|
Text2
|
|
txtAnswer1
|
Delete existing text
|
Text3
|
|
txtAnswer2
|
Delete existing text
|
Text4
|
|
txtAnswer3
|
Delete existing text
|
Check1
|
|
chkAnswer1
|
Delete existing caption
|
Check2
|
|
chkAnswer2
|
Delete existing caption
|
Check3
|
|
chkAnswer3
|
Delete existing caption
|
Exit
|
|
cmdExit
|
|
Coding
:
You've now finished
setting up the properties and names for
the controls on our form and we're now
able to make it do something!!
We need to write code to instruct the
Calculate command button to respond to a
mouse click. The program should then look
at the values entered into the Price and
Quantity text boxes, multiply them
together and put the answer in the Total
Price text box.
You will write an event procedure called
cmd_Calculate_Click. The name of this
procedure consists of the object's name
(cmdCalculate), the underscore character
(_) and the type of event (Click).
Don't worry if this sounds complicated.
Visual Basic will automatically create
the procedure names for you!!
When is a Text
box not a Text box?
All data entry in Visual
Basic is treated as text, even if numbers
are entered. Unlike older versions of
Basic, Visual Basic will sometimes
recognise that numbers have been entered
and will allow them to be used in
calculations.
However, care needs to be taken when
making assumptions about numbers entered
into text boxes. At a later stage, we
will make sure the user isn't allowed to
enter letters by mistake in a text box
that should contain a number, and that
numbers are treated correctly by Visual
Basic.
Task Three :
1. Double click on the
command button named
"cmdCalculate" (caption
"Calculate"). The procedure
name and End Sub is automatically
entered:
Sub cmdCalculate_Click()
End Sub
2.
Complete the event procedure by entering
the calculation for Total Price:
Sub cmdCalculate_Click()
txtTotalPrice
= txtPrice * txtQuantity
End Sub
This will
take the value entered in the Price text
box (txtPrice), multiply it (note the *)
by the value entered in the Quantity text
box (txtQuantity) and place the answer in
the Total Price text box (txtTotalPrice).
3. Click on the Start Program shortcut on
the toolbar.
4. Type in Headphones for the Item, enter
3.50 for the Price and 2 for the
Quantity.
5. Click on the Calculate button and the
answer 7 should appear in the Total Price
text box. We'll worry about £ signs and
decimal places later.
6. Try entering other values for Price
and Quantity and calculating the Total
Price.
7. Click on the Stop Program shortcut on
the toolbar.
8. We need a better way of stopping the
program, one that will be available as
part of the form. Double Click on the
button called "cmdExit"
(Caption "Exit")
9. The programming command to stop the
program is End. Enter this into the event
procedure for cmdExit_Click:
Sub cmdExit_Click()
End
End Sub
10. Run
the program again using the Start Program
shortcut, try various calculations and
then click the Exit button to finish.
11. Save your project.
Exercise Three
:
1. Change the Caption of
the Total Price label to Sub Total.
Change the name of txtTotalPrice to
txtSubTotal.
2. Create a new label with the caption
'VAT'. Create a new text box withe the
name txtVAT and clear its text property.
3. Edit the cmdCaltulate_Click() event
procedure to work out the sub total
(txtSubTotal=txtPrice*txtQuantity) and an
extra line to calculate the VAT
(txtVAT=txtSubTotal * 17.5 / 100).
4. Create a new label and text box for
'Price Including VAT' and add an extra
line to the cmdCalculate_Click()
procedure to calculate a value for this
new text box.
Save your work and run your program with
various values.
Page [<<
PREV] 1
2 3 4
5 6
[NEXT
>>]
Back to Tutorials
- Main
|